New Jersey, USA
-
Location
-
Phone
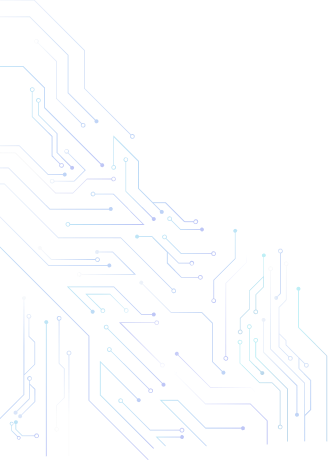
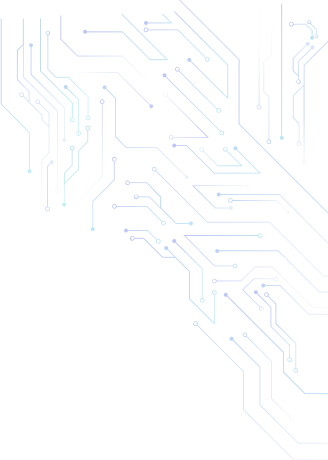
How to Optimize Your Mobile App for Performance
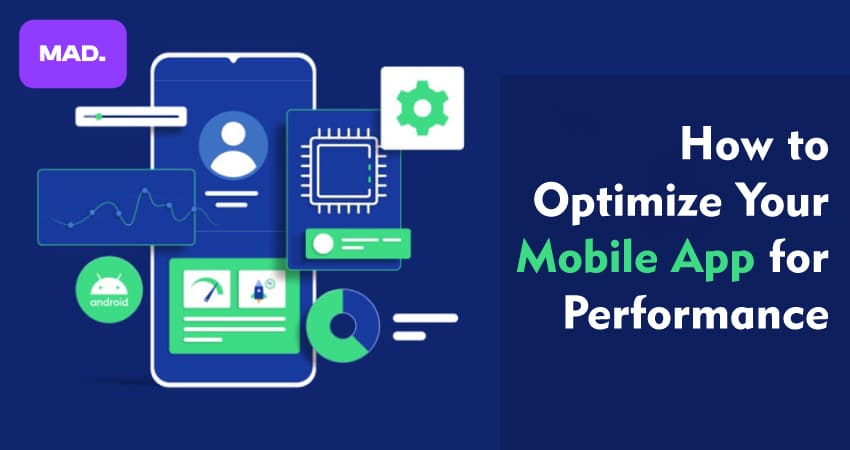
To optimize your mobile app for performance, focusing on speed, responsiveness, and efficient resource use is key. This involves techniques that minimize app lag, reduce load times, and enhance the user experience. Here’s a comprehensive guide covering the essentials of mobile app optimization:
“Optimizing mobile app performance involves reducing app size, improving code efficiency, managing memory, and minimizing network calls. Key strategies include removing unused resources, using vector graphics, and implementing lazy loading to load only necessary data. Efficient memory management, caching, asynchronous loading, and battery optimization further improve responsiveness and speed. Monitoring tools like Firebase Performance Monitoring and Android/iOS profilers are essential for detecting performance bottlenecks early. By integrating these techniques throughout development, Mobile App Development (MAD) practitioners can create apps that perform well across devices, ensuring user satisfaction and long-term app success in a competitive market.”
1 Minimize App Size
A large app size can affect performance and discourage downloads due to data usage and storage concerns. Here are a few ways to reduce app size:
- Remove Unused Resources: Over time, your app may accumulate resources like images, audio files, or other assets that are no longer in use. Periodically audit and remove these.
- Use Vector Graphics: When feasible, think about utilizing vector graphics in place of high-resolution pictures. Vector files are scalable, lightweight, and look great on any screen size.
- Code Minification and Obfuscation: ProGuard (for Android) or Xcode’s built-in optimization can help reduce your app’s size by compressing code and resources.
2 Optimize Code Efficiency
Inefficient code can significantly degrade app performance. Here’s how to ensure your code is clean and optimized:
- Reduce Code Complexity: Avoid deep nested loops and long execution paths, which can slow down processing. Focus on keeping code lean and refactoring regularly.
- Optimize Data Structures and Algorithms: Choose the most efficient data structures for your app’s needs. Using the correct algorithms for sorting, searching, or filtering can drastically improve response times.
- Lazy Loading: Only load data when necessary. For example, load images as the user scrolls through a list rather than all at once.
3 Efficient Memory Management
Memory usage is a major concern in mobile apps, as Android and iOS have limitations. Memory leaks can result in app lag, crashes, or even the OS killing the app.
- Avoid Memory Leaks: As soon as a resource is no longer required, make sure it is released appropriately. Memory profiling tools like Xcode Instruments or Android Studio Profiler can help identify leaks.
- Use Weak References: In Java, weak references can help prevent memory leaks by allowing objects to be garbage-collected if they’re no longer in use.
- Optimize Bitmap Usage: Use in-memory caching and avoid loading high-resolution images in their full size unless necessary. Tools like Glide or Picasso can help manage image loading effectively.
4 Reduce Network Calls
Network operations are one of the primary causes of slow performance. Here’s how to minimize the impact:
- Batch Network Requests: Instead of making multiple network requests, try batching them into a single call.
- Use Caching: Cache data whenever possible to reduce the number of network calls. This is especially useful for data that doesn’t change frequently, like profile pictures or static content.
- Reduce Data Transfer: Compress data before sending it over the network. JSON is lighter than XML, for example, so it’s often a better choice for data serialization.
5 Implement Background Threading
Handling long-running or resource-heavy tasks in the main UI thread can cause the app to become unresponsive, resulting in a poor user experience.
- Use Background Threads: Shift heavy computations, network calls, and file I/O operations to background threads. This keeps the UI responsive.
- Android WorkManager and iOS Background Tasks: For sporadic background work, use system-provided APIs like iOS's Background Tasks or Android's WorkManager.
6 Optimize the User Interface (UI)
A smooth UI experience is critical for user satisfaction and app success.
- Reduce Layout Complexity: Deep layout hierarchies can slow down rendering. Flatten complex layouts, and avoid using too many nested views. Use ConstraintLayout (Android) or StackViews (iOS) to simplify complex layouts.
- Optimize Animations: Avoid overusing animations as they can increase CPU and memory usage. Make sure any animations you use are lightweight and optimized. Use the hardware-accelerated graphics rendering if possible.
- Pre-compute Layouts: If you have complex layout calculations, try to pre-compute and cache them, rather than recalculating on every render.
7 Database Optimization
Apps that heavily depend on data, especially offline applications, need optimized databases to manage and retrieve information efficiently.
- Index Frequently Queried Columns: Indexing columns that are frequently queried improves retrieval speed in SQL-based databases.
- Use Proper Database Libraries: Libraries like Room (Android) or Core Data (iOS) are optimized for mobile devices and can handle data efficiently.
- Minimize Database Writes: Writing data to the database frequently can be resource-intensive. Instead, batch writes whenever possible.
8 Reduce Battery Consumption
attery usage is a significant factor that impacts user experience. Users may abandon an app that drains their battery quickly.
- Limit Background Processes: Minimize background tasks and only run them when necessary. For instance, avoid running location services continuously if the app doesn’t require it.
- Optimize GPS and Location Services: Location services are among the most battery-consuming resources. Consider using less frequent location updates or only accessing locations when needed.
- Efficient Network Use: Large amounts of data transfer and frequent network calls can drain battery life. Implement caching and offline data strategies where possible to minimize unnecessary data transfers.
9 Optimize for Different Screen Sizes and Orientations
Your app should look good and function smoothly across various devices and screen sizes.
- Use Adaptive Layouts: Make sure your app's user interface (UI) adapts to various screen sizes and orientations by utilizing layouts that are based on constraints (such as Auto Layout in iOS or ConstraintLayout in Android).
- Responsive Font and Image Sizes: Use scalable vector images and responsive fonts that adapt to different screen densities and resolutions.
10 Measure Performance with Tools
Monitoring and evaluating performance at each development phase will help you catch and fix issues before they affect the user experience.
- Android Profiler: Android Studio includes a suite of profiling tools for memory, CPU, and network, which can help track down performance bottlenecks.
- Xcode Instruments: Xcode offers Instruments for iOS app performance profiling, with tools for measuring memory usage, CPU load, and more.
- Third-Party Tools: Consider using tools like Firebase Performance Monitoring or Flurry Analytics to get real-time insights into app performance issues.
11 Optimize the Startup Time
Fast startup times can significantly improve user satisfaction. Here are some tips to minimize your app’s launch time:
- Lazy Load Non-Essential Components: Only load essential components on startup and delay loading other parts until the user needs them.
- Reduce Initial Network Calls: Minimize network calls on the startup sequence. If your app requires data from a server, consider loading cached data first and refreshing it in the background.
- Warm Start Optimizations: Once your app is in the background, ensure that it can resume quickly when the user returns to it.
12 Implement Efficient Logging Practices
While logging is essential for debugging, excessive logging can slow down your app and increase storage use.
- Log Only When Necessary: Limit logging to essential events. Avoid logging sensitive information, which can also create security risks.
- Disable Logging in Production: Many logging libraries provide options to disable logging in production, which can help conserve resources and enhance performance.
13 Use Caching Strategically
Caching can significantly reduce load times and data transfer costs by reusing previously loaded resources.
- In-Memory Cache: Frequently accessed data can be stored in memory for quick access. However, be mindful of memory limitations.
- Disk Cache: Store large or less frequently accessed data on disk. This is especially useful for storing images or other media assets.
- Implement Cache Expiration: Set expiration policies for cached data to ensure the app isn’t using stale data.
14 Monitor and Optimize Network Latency
Network latency can cause noticeable delays in app performance, especially in apps that rely heavily on data from remote servers.
- Use Data Compression: Compressing data before it’s sent over the network can help reduce latency and bandwidth usage.
- Implement Retry and Timeout Logic: If a network request fails, it should retry up to a certain limit, while also ensuring timeouts to prevent endless loading.
- Prioritize Requests: Assign priority to essential requests and defer non-essential requests, reducing the load on your server and network.
15 Ensure Backward Compatibility
Supporting older devices and operating systems can improve your app’s reach and performance on various devices.
- Use Device Compatibility Libraries: Libraries like AndroidX and backport libraries can help maintain backward compatibility without bloating the app.
- Conditional Feature Usage: Use features only on supported devices. Avoid resource-intensive features on devices with limited processing power.
16 Enable Asynchronous Loading
Asynchronous loading allows the app to continue functioning while loading data or resources in the background.
- Use Async Tasks Wisely: Instead of blocking the main thread, load data asynchronously so the user can still interact with the app while content is loading.
- Coroutines and Futures: On Android, Kotlin Coroutines can be used to perform asynchronous tasks efficiently. iOS offers Grand Central Dispatch (GCD) for handling asynchronous work.
17 Optimize Push Notifications
Push notifications can impact app performance and user experience if not handled efficiently.
- Control Frequency: Excessive push notifications can annoy users and lead them to uninstall the app. Limit push notifications to essential updates or messages.
- Efficient Background Sync: Use push notifications to trigger lightweight background syncs rather than downloading large data chunks.
18 Use Lightweight Libraries and Frameworks
Heavy libraries can slow down your app and increase its size. Evaluate each library you add to ensure it’s necessary.
- Use Only Essential Libraries: Avoid unnecessary libraries that add extra weight and potential overhead.
- Evaluate Library Performance: Opt for libraries that are optimized for mobile performance, or explore lightweight alternatives.
19 Regularly Test on Real Devices
Testing only in an emulator can lead to performance discrepancies when the app runs on real devices.
- Diverse Testing Devices: Test on a variety of devices with different performance capabilities, screen sizes, and OS versions.
- Field Testing: Testing in real-world scenarios can help identify unexpected issues related to network variability, GPS accuracy, and battery usage.
20 Implement Performance Monitoring for Future Optimization
Continuous monitoring provides insights into areas where performance could improve.
- Crash and Performance Analytics: Use tools like Firebase Crashlytics or Sentry for real-time crash reporting and user experience insights.
- Measure Key Performance Indicators (KPIs): Track app load times, network request speeds, and resource consumption to gauge performance over time.
Conclusion
In Mobile App Development (MAD), optimizing for performance is paramount in delivering a smooth, engaging, and responsive user experience. By focusing on techniques like minimizing app size, optimizing code efficiency, managing memory usage, and implementing asynchronous loading, you address potential bottlenecks that could slow down the app or drain device resources. Each optimization technique, from reducing network calls to ensuring backward compatibility, helps build a robust app that performs well across a range of devices and conditions.
Performance monitoring and testing on real devices ensure that potential issues are identified and addressed early, while tools like Firebase Performance Monitoring and Android/iOS profiling tools offer actionable insights. With these optimizations, MAD practitioners can enhance speed, responsiveness, and efficiency, setting their app up for success in an increasingly competitive marketplace. By embedding performance optimization into every stage of development, you can deliver a mobile app that not only meets user expectations but also fosters loyalty and satisfaction.
MAD is a top app development company in the USA, a high-performance low-code platform that enables rapid and easy mobile app development compared to traditional coding methods.